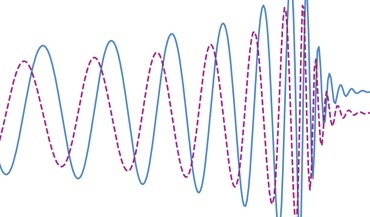
Symmetries of spacetime infinitely far away from gravitational fields may hint at new laws of nature
This page explains how to work with MATLAB R2015a (8.5.0.197613) 64-bit (glnxa64), when connected to one of LIGO's clusters: CIT, AEI, etc. The user interface is UNIX, and we consider how to work with MATLAB from the command line.
For information on how to run MATLAB in Python see: Useful python commands.
MATLAB utilises different functions, some of them are buit-in, while others can be called from the outside world, or written by user. In order to "tell" MATLAB about those other functions one needs to configure startup.m file. This is just the text file in the folder from which MATLAB is going to be launched.
We can use MATLAB in three main ways:
matlab -nodesktop -nodisplay
, and then type commands one-by-one.command + Enter
.When MATLAB gives you error when running a certain script, it provides user with a line number in the script where error occurred. To see what causes the error, and to fix it, we can stop the program execution just before the error, by inserting a keyboard;
command just before the problematic line of code.
Introducing a keyboard;
command in the script pauses the execution at the line where this command is inserted. In this regime it is possible to see what variables are, and how the script would respond to various commands.
dbquit
to exit the code executiondbcont
to continue the code execution up to a next keyboard insertionSometimes we need to compile MATLAB code. In example, when we need to run it on Condor (parallel computing), or to run it from the UNIX shell, without launching MATLAB.
I recommend to use my code for compilation on the CIT: /home/boris.goncharov/common/lib/mcc_fast.m
(adapted from Eric Trane's code)
Or to create a following script:
function mcc_fast(fname)
% example usage: mcc_fast('preproc');
% inclide repositories that your compiled script uses.
% Each subdirectory should be included separately.
stamp2 = {'/home/boris.goncharov/shortcuts/stamp2/src/', ...
'/home/boris.goncharov/shortcuts/stamp2/src/algorithms/stochtrack', ...
'/home/boris.goncharov/shortcuts/stamp2/src/img', ...
'/home/boris.goncharov/shortcuts/stamp2/src/gpu_tools', ...
'/home/boris.goncharov/shortcuts/stamp2/src/inj', ...
'/home/boris.goncharov/shortcuts/stamp2/src/tools', ...
'/home/boris.goncharov/shortcuts/stamp2/src/misc', ...
'/home/boris.goncharov/shortcuts/stamp2/src/misc/utilities', ...
'/home/boris.goncharov/shortcuts/stamp2/input/', ...
'/home/boris.goncharov/shortcuts/stamp2/src/crosscorr'
};
% start off by requiring stamp code
comp_cmd = 'mcc -v -N';
for ii=1:numel(stamp2)
comp_cmd = [comp_cmd ' -I ' stamp2{ii}];
end
% path to signals toolbox
sigs = '/ldcg/matlab_r2013a/toolbox/signal/signal';
stats = '/ldcg/matlab_r2013a/toolbox/stats/stats';
% add parallel without java to run gather()
% this is needed for stochtrack on a single core
parallel = '/ldcg/matlab_r2013a/toolbox/distcomp/parallel/';
% add this to run stochtrack on a single gpu
gpu1 = '/ldcg/matlab_r2013a/toolbox/distcomp/gpu/';
gpu2 = '/ldcg/matlab_r2013a/toolbox/distcomp/array';
% in order to use padarray.m (Thanks, Michael)
images = '/ldcg/matlab_r2013a/toolbox/images/images/';
% distributed computing (THIS DOES NOT WORK...without java)
%dist = '/ldcg/matlab_r2013a/toolbox/distcomp/';
% java
%java = '/ldcg/matlab_r2013a/toolbox/javabuilder/javabuilder';
% -I flags
includes = { sigs stats parallel images gpu1 gpu2 };
% -R flags
options = { '-nodisplay','-nojvm','-singleCompThread' };
% compilation command includes
for ii=1:numel(includes)
comp_cmd = [comp_cmd ' -I ' includes{ii}];
end
% ...options
for ii=1:numel(options)
comp_cmd = [comp_cmd ' -R ' options{ii}];
end
% ...add -m flag
comp_cmd = [comp_cmd ' -m ' fname];
% compile
fprintf('%s\n',comp_cmd);
eval(comp_cmd);
% remove annoying files
system(['rm mccExcludedFiles.log readme.txt run_' fname '.sh']);
return
Also one can compile the script by mcc -m code_name
, but it adds a lot of unnescessary libraries in the compiled program, raising its RAM requirements.
Symmetries of spacetime infinitely far away from gravitational fields may hint at new laws of nature
Is the common-spectrum process observed with pulsar timing arrays a precursor to the detection?